Setting up a microservices architecture with service discovery and registration (e.g., Consul)?
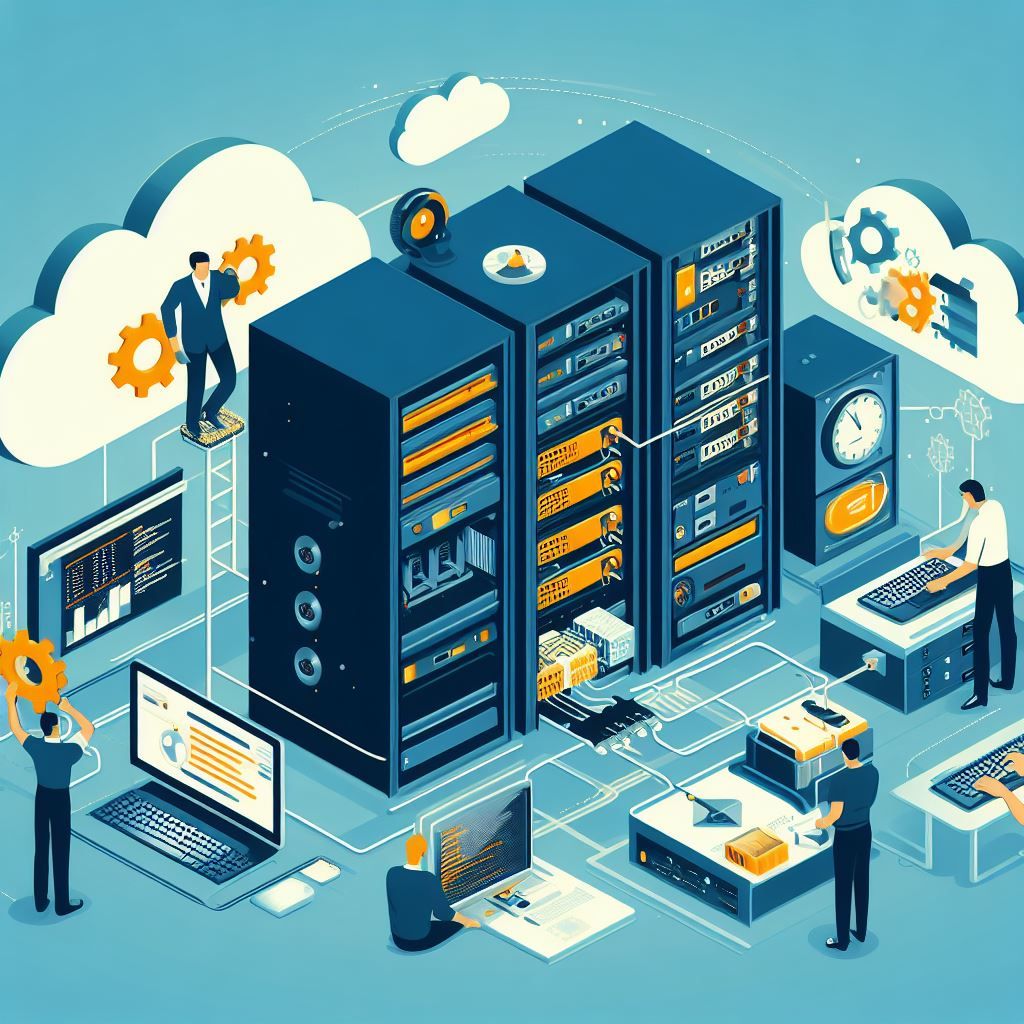
Setting up a microservices architecture with service discovery and registration, such as using Consul, involves several key steps. Service discovery and registration are crucial components that help microservices locate and communicate with each other dynamically. Below is a general guide on how you can set up such an architecture:
- Define Your Microservices:
Clearly define the microservices that make up your system and their respective functionalities. Each microservice should have a well-defined API. - Containerization:
Consider using containerization technologies like Docker to package your microservices along with their dependencies. This ensures consistency across different environments. - Orchestration:
Choose an orchestration tool such as Kubernetes or Docker Swarm to manage and deploy your containers. Orchestration simplifies the process of deploying, scaling, and managing containerized applications. - Service Discovery:
Integrate a service discovery tool like Consul into your architecture. Consul provides a way for services to register and discover each other dynamically. - Install and Configure Consul:
Set up a Consul cluster. You can either run Consul as a standalone binary, deploy it as a container, or use a configuration management tool. Configure Consul agents on each node where your microservices will run.bashCopy code# Example command to start a Consul agent
consul agent -dev - Service Registration:
Modify your microservices to register themselves with Consul when they start. This involves making an API call to the Consul agent to announce the service's availability.bashCopy code# Example registration using curl
http://localhost:8500/v1/agent/service/register
curl -X PUT -d '{"ID": "my-service-1", "Name": "my-service", "Port": 8080}' - Service Discovery:
Update your microservices to use Consul's DNS or HTTP API to discover the location of other services. Instead of hardcoding IP addresses and ports, services can dynamically query Consul for the current service locations.bashCopy code# Example DNS query
nslookup my-service.service.consul - Health Checks:
Configure health checks for your microservices in Consul. This ensures that Consul can identify healthy and available instances of each service. - Load Balancing:
If needed, set up load balancing for incoming requests. Consul can provide information about multiple instances of a service, and load balancing mechanisms can distribute traffic among them. - Monitoring and Logging:
Implement monitoring and logging to keep track of the health and performance of your microservices. Tools like Prometheus and Grafana can be integrated into your architecture. - Security:
Implement security measures, such as encrypting communication between microservices and securing access to Consul. Consul supports encryption and ACLs (Access Control Lists) to enhance security. - Documentation:
Document your service discovery and registration mechanisms, including how services should register, how to perform health checks, and how to consume the discovered services.
Remember that the specifics of implementation may vary based on the technologies you choose and the requirements of your application. Regularly test and iterate on your setup to ensure its reliability and scalability.