A Guide to Implementing Microservices Architecture on Cloud Servers
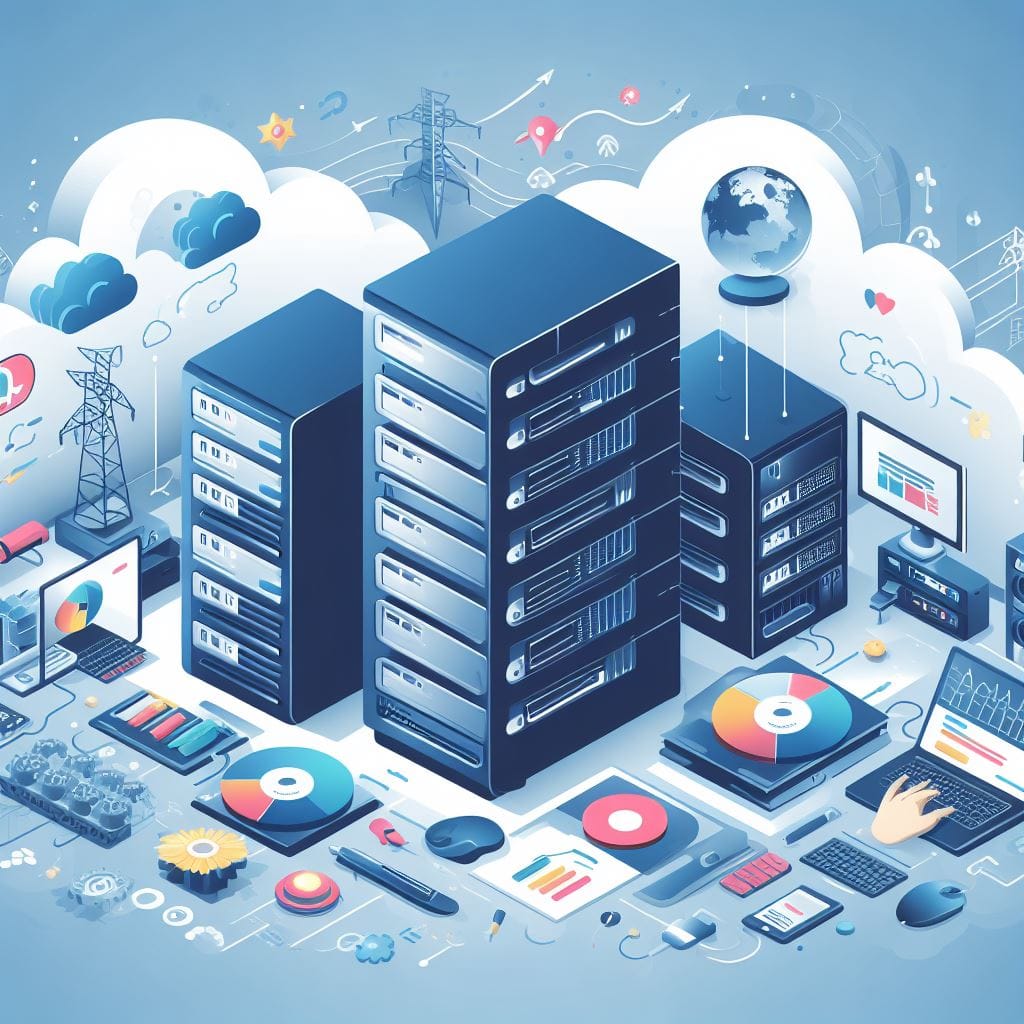
Implementing a microservices architecture on cloud servers involves breaking down a large application into smaller, loosely coupled services that can be independently developed, deployed, and scaled. This approach can offer benefits like improved scalability, flexibility, and maintainability. Below is a guide to help you implement microservices on cloud servers:
- Define Your Microservices:
- Identify the different components or functionalities of your application that can be broken down into individual services. Each service should have a specific and well-defined purpose.
- Select a Cloud Provider:
- Choose a cloud provider that aligns with your requirements. Popular options include AWS (Amazon Web Services), Azure, Google Cloud Platform (GCP), and others. Different providers offer various services that can be leveraged in your microservices architecture.
- Set Up a Development Environment:
- Create a development environment that allows your team to work on the individual microservices. Each service should be developed and deployed independently.
- Decide on Communication Protocols:
- Choose how your services will communicate with each other. Common methods include HTTP/HTTPS (RESTful APIs), message queues (like RabbitMQ, Kafka), or gRPC for more performance-intensive applications.
- Containerization and Orchestration:
- Containerize your microservices using tools like Docker. This ensures consistency between development, test, and production environments.
- Use a container orchestration platform like Kubernetes to manage and deploy your containerized services at scale.
- Implement API Gateways:
- An API gateway acts as a front door to your microservices. It handles routing, authentication, and other cross-cutting concerns. Popular options include Amazon API Gateway, NGINX, or building a custom solution.
- Service Discovery and Load Balancing:
- Implement a service discovery mechanism to dynamically locate services at runtime. Tools like Consul, Eureka, or Kubernetes' built-in service discovery can be used.
- Set up load balancing to distribute incoming requests evenly across multiple instances of a service. This ensures high availability and scalability.
- Database Per Service:
- Each microservice should have its own database or data store that is specific to its needs. This minimizes dependencies between services and allows for independent scaling and maintenance.
- Security and Authorization:
- Implement proper security measures like HTTPS, authentication, and authorization for your services. Consider using tools like JWT (JSON Web Tokens) or OAuth for authentication.
- Monitoring and Logging:
- Set up monitoring tools to track the health and performance of your microservices. Use tools like Prometheus, Grafana, or cloud-native solutions provided by your chosen cloud provider.
- Implement centralized logging to collect and analyze logs from all your services. Tools like ELK stack (Elasticsearch, Logstash, Kibana) or cloud-native logging services can be used.
- Fault Tolerance and Resilience:
- Design your microservices to be resilient to failures. Implement practices like circuit breakers, retries, and timeouts to handle service failures gracefully.
- Automated Deployment and CI/CD:
- Set up a continuous integration and continuous deployment (CI/CD) pipeline to automate the deployment of your microservices. Use tools like Jenkins, GitLab CI/CD, or cloud-native solutions.
- Scalability and Auto-scaling:
- Leverage cloud services to dynamically scale your microservices based on demand. Configure auto-scaling rules to add or remove instances as needed.
- Testing and Quality Assurance:
- Implement unit tests, integration tests, and end-to-end tests for each microservice. Consider using tools like JUnit, Postman, or Selenium for automated testing.
- Documentation and Communication:
- Document the APIs, communication protocols, and any special considerations for each microservice. Foster strong communication and collaboration within your development team.
Remember that implementing a microservices architecture is a complex task, and it's important to continuously monitor and iterate on your architecture as your application evolves. Additionally, consider the trade-offs involved, such as increased operational complexity compared to a monolithic architecture.