A Guide to Building Cloud-Native Applications with Containers and Microservices
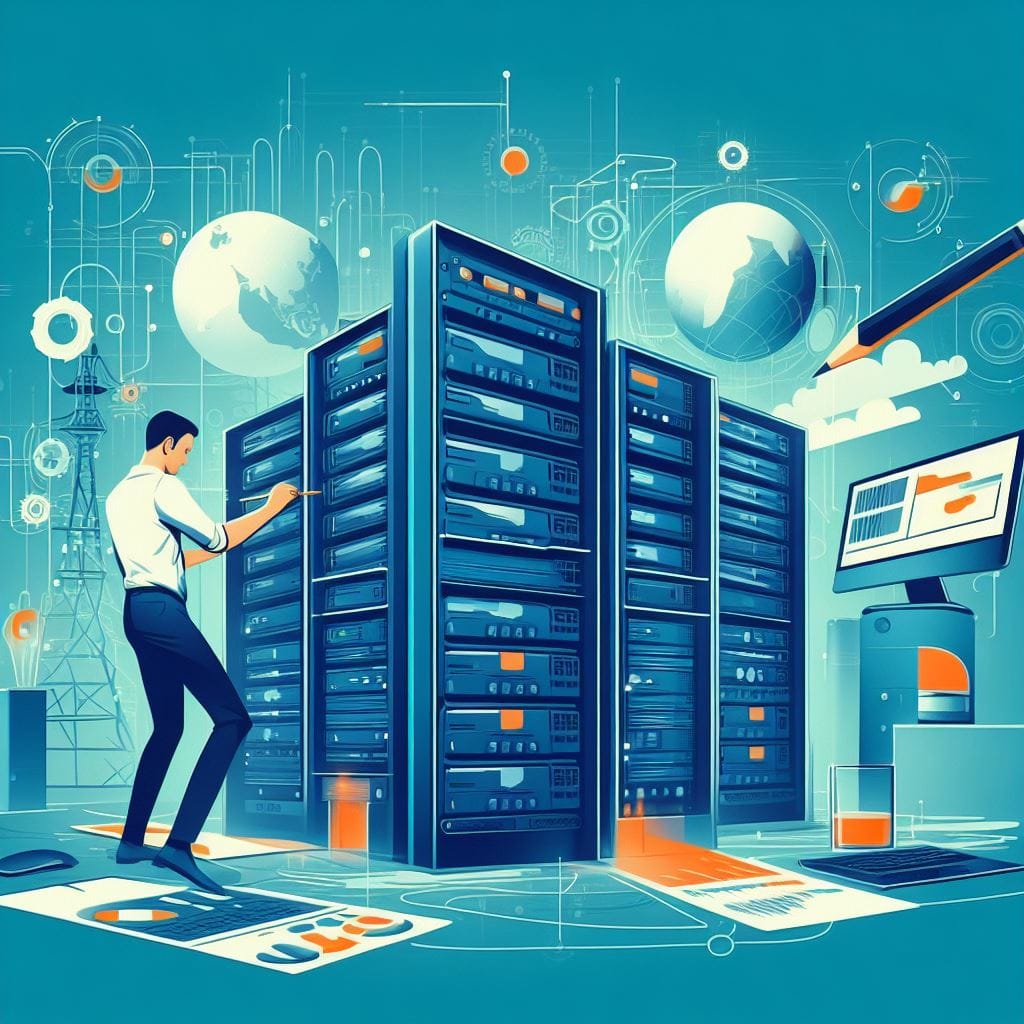
Building cloud-native applications with containers and microservices involves designing and developing applications in a way that leverages the benefits of cloud computing, such as scalability, flexibility, and resilience. Here's a guide to help you get started:
1. Understand the Principles of Cloud-Native Development:
- Microservices Architecture: Break down your application into smaller, loosely coupled services that can be developed, deployed, and scaled independently.
- Containerization: Use container platforms like Docker to package applications and their dependencies, ensuring consistency across different environments.
- Orchestration: Utilize tools like Kubernetes to automate the deployment, scaling, and management of containerized applications.
- DevOps and CI/CD: Implement continuous integration and continuous deployment practices to streamline development, testing, and deployment processes.
- Resilience and Fault Tolerance: Design applications to handle failures gracefully and recover quickly.
2. Select the Right Technology Stack:
- Container Runtime: Docker is a popular choice, but other runtimes like containerd and rkt are also available.
- Orchestration Platform: Kubernetes is the industry standard, but alternatives like Docker Swarm and Amazon ECS also exist.
- Service Discovery and Load Balancing: Tools like Kubernetes Services, Consul, or Istio help with service discovery and load balancing.
- Monitoring and Logging: Use tools like Prometheus for monitoring and ELK stack (Elasticsearch, Logstash, Kibana) for logging.
3. Design and Architect Your Microservices:
- Decompose your application: Identify the different components or functionalities that can be isolated into microservices.
- Define clear API contracts: Establish well-defined interfaces for communication between microservices, often using RESTful APIs or gRPC.
- Ensure Data Independence: Each microservice should have its own database or data store, and communication between services should be through APIs.
- Handle Cross-Cutting Concerns: Address concerns like authentication, authorization, logging, and monitoring in a consistent way across services.
4. Containerizing Your Application:
- Write Dockerfiles: Create Dockerfiles to define how your application and its dependencies should be packaged into a container.
- Use Multi-Stage Builds: Optimize Dockerfiles to minimize image size and improve security.
- Leverage Container Registries: Store and manage your container images in registries like Docker Hub, Google Container Registry, or Amazon ECR.
5. Implement CI/CD Pipelines:
- Version Control: Use a version control system like Git to manage your source code.
- Automated Testing: Include unit tests, integration tests, and end-to-end tests in your CI/CD pipeline.
- Continuous Deployment: Automate the process of deploying new versions of your application to your target environment.
6. Deploy and Manage with Orchestration:
- Define Kubernetes Manifests: Use YAML files to describe the desired state of your application, including services, deployments, and pods.
- Set Up Configurations and Secrets: Manage environment-specific configurations and sensitive information using ConfigMaps and Secrets.
- Implement Health Checks and Readiness Probes: Ensure that Kubernetes can monitor the health of your application and determine when it's ready to receive traffic.
- Scale Horizontally: Use Kubernetes' scaling features to handle increased load.
7. Implement Monitoring and Observability:
- Set up Metrics and Alerts: Use tools like Prometheus to collect metrics and set up alerts for abnormal behavior.
- Logging and Tracing: Aggregate logs for easier troubleshooting and implement distributed tracing for end-to-end visibility.
- Monitor Resource Utilization: Keep an eye on CPU, memory, and storage usage to ensure efficient resource allocation.
8. Handle Service Discovery and Load Balancing:
- Use Kubernetes Services: Create Services to abstract away the underlying IP addresses of your microservices.
- Implement Load Balancing: Kubernetes Services provide built-in load balancing capabilities.
9. Security Best Practices:
- Implement Network Policies: Define network policies to control traffic between pods.
- Use Role-Based Access Control (RBAC): Limit access to Kubernetes resources based on roles and permissions.
- Secure Secrets Management: Use Kubernetes Secrets or external secret management tools to handle sensitive information.
- Scan Container Images for Vulnerabilities: Use tools like Clair or Trivy to scan container images for security issues.
10. Continuously Iterate and Improve:
- Collect and Analyze Metrics: Use feedback loops to identify areas for improvement and optimize your architecture and processes.
- Embrace Cloud-Native Technologies: Stay up-to-date with new technologies and best practices in the cloud-native ecosystem.
Remember, building cloud-native applications is an iterative process. Embrace a culture of continuous improvement, and be open to adapting your architecture and practices as your application evolves.